Create an animation that shows a clock for aliens. The clock cannot display time tied to the rotation of the Earth - years, months, days, hours, minutes, etc. Instead create your own system of time - however large or small. If the clock is no longer tied to celestial movement - how else can you express the passing of time? Would the time be continuous or discrete? Cyclical or unidirectional? How would you divide it into sub-elements and what visual tools would you use to represent distinctions: color, shape, size, order? Use arrays and loops. Use rotation with the transformation matrix. Use either trigonometric functions or intervals (or both if need be).
After reviewing the brief, I envisioned designing an innovative clock as an alien creature itself—specifically, a chameleon-like being that communicates and measures time through its shifting colors. This clock is inspired by the dynamic nature of the alien lifeform. It acts as a captivating rotating color wheel that cycles through the entire spectrum of hues. This design mimics the vibrant display of colors seen on the creature's skin, reflecting its ability to change colors.
As the alien shifts through its colors, it creates a mesmerizing visual experience that draws the viewer in. Every time the creature comes to rest on the color red, it serves as a signal to the aliens that a full day has passed in their world. In this way, the clock allows the aliens to perceive the passage of time through color changes.

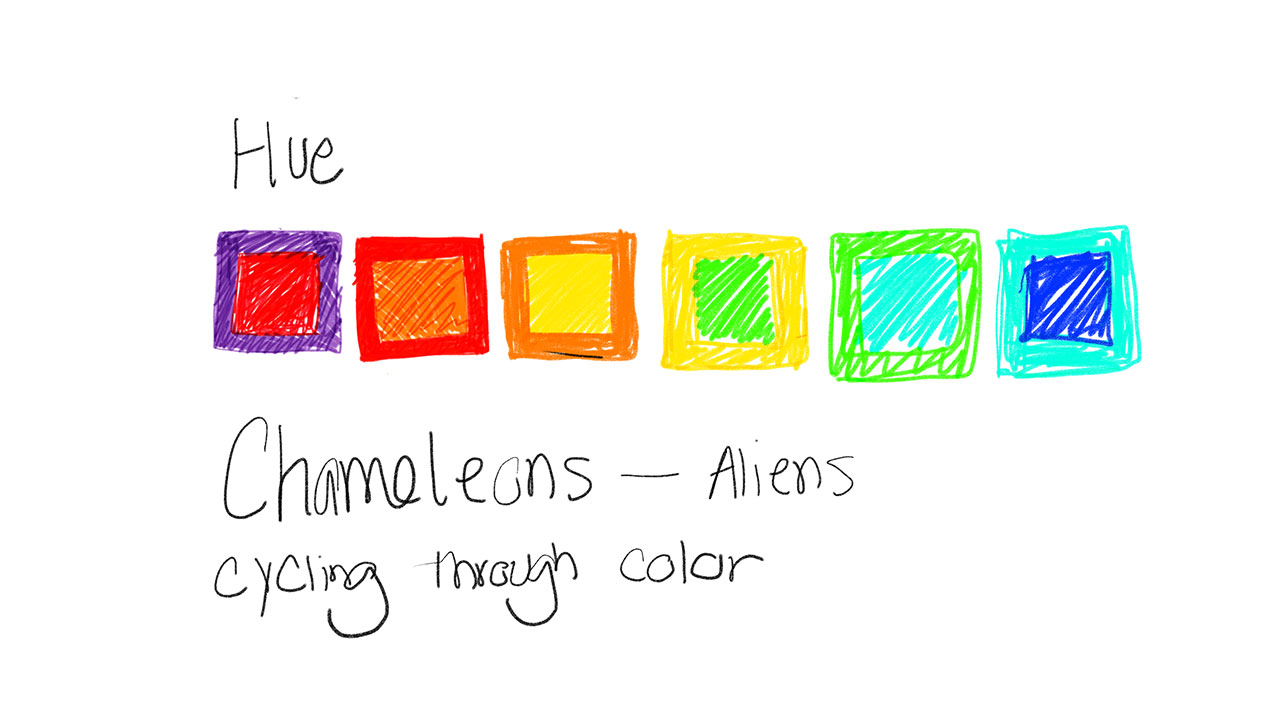
I felt a surge of excitement to start designing an alien clock. I began brainstorming and discussing my ideas with my peers. I recalled a film I had watched called ‘NOPE,’ where the alien creature captivated me. This inspired me to conceptualize the alien itself as a clock. I realized that aliens might communicate visually, which opened up new possibilities for my design.
Using the code that Maxim provided in class, I've decided to use it as the foundation for my project. This will serve as the starting point for my own generative animation. I can’t just jump into coding; I need to reference someone else's work to understand the concepts and approach involved in tackling this challenge.